Setting up your first Next.js project
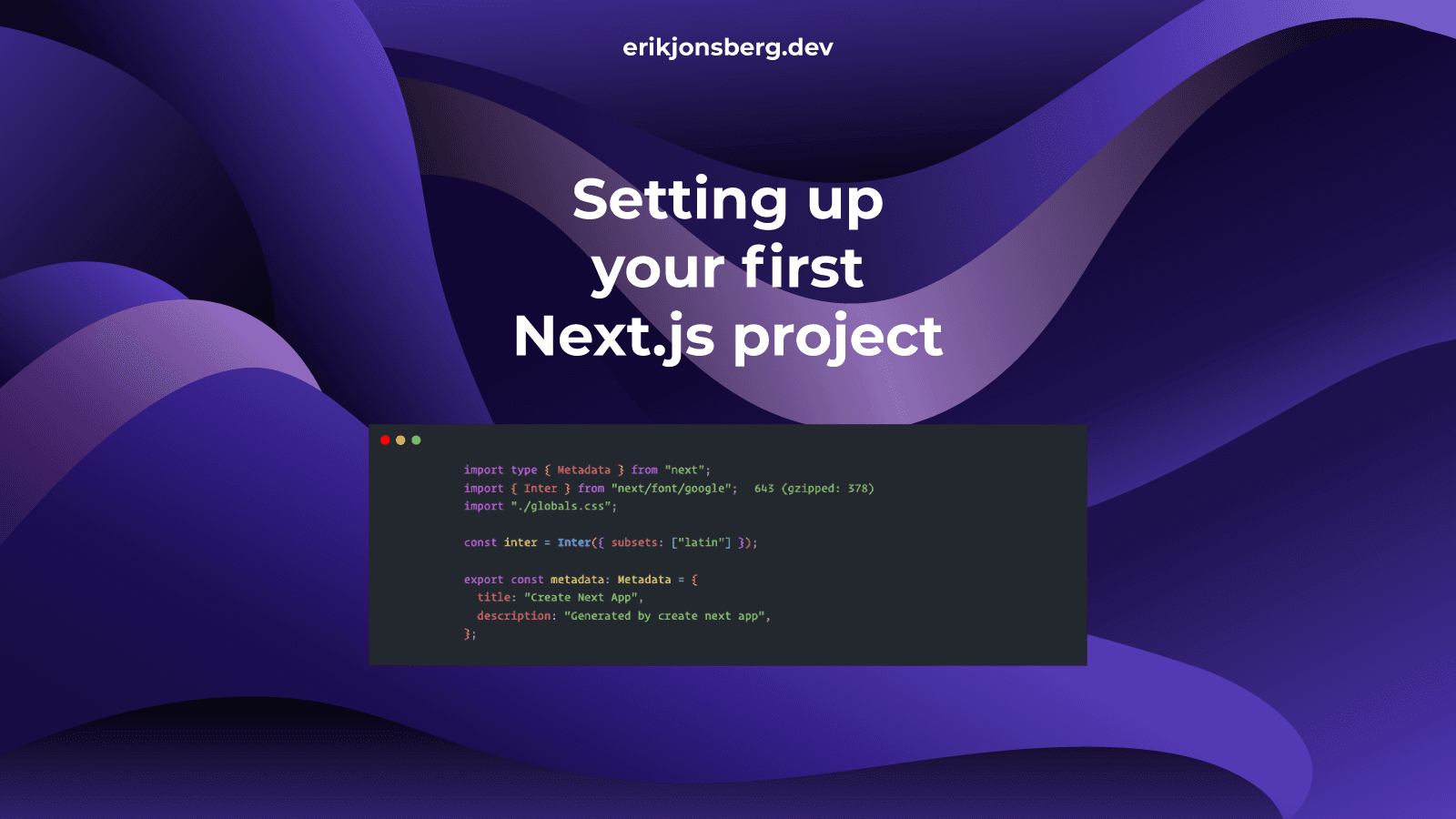
Before starting, ensure you have Node.js version 18 or higher. You can check your version by typing node -v in the command line. If you don't have it, you can download it here.

The Node.js team recommends using the Long-Term Support (LTS) version for most projects because it's more stable and has guaranteed support for 30 months. While the current version has more features, it's only supported for six months and is potentially less stable. I've used it in past projects, and it's hard to maintain because it gets updated frequently, and many of the changes break your project.
You can install both versions to test the latest features but switch to a stable version for production. Then, you can install the Node Version Manager (NVM). NVM lets you switch between versions with a simple command: nvm use <version>
. You can also use it to install and delete multiple Node versions, which can be helpful when working with legacy code.
Now that you have the latest stable version of Node.js installed on your machine, you're ready to create your first Next.js app.
Open a window in Visual Studio Code, start a new terminal, and cd into the directory where you want to create your app.
$ cd my-next-project
Next, enter the following command:
$ npx create-next-app@latest
The installer will ask you a series of questions about your app.

Here's a breakdown of the reasoning behind each choice:
- What is your project named? You can name it whatever you want — the default is my-app
- Would you like to use TypeScript? Choose yes for several reasons:
- Code that's easy to read and maintain.
- More intelligent autocomplete suggestions from your IDE.
- Ability to catch errors as they happen instead of waiting for runtime — say goodbye to unknown type errors.
- Typescript helps enforce coding standards and allows team members to see what others are working on.
- Would you like to use ESLint? Choosing yes installs ESLint and several next and react-specific linter plugins.
- Would you like to use Tailwind CSS? This is a personal preference. You can use whichever CSS library you want, like MUI or Bootstrap, and Next.js has built-in support for CSS Modules.
- Would you like to use
src/
directory? Another personal preference. Using src/ can help you keep your project better organized, but it's optional. - Would you like to use App Router? An emphatic yes! The app router offers a more intuitive API, simplified data fetching, and default server-side rendering.
- Would you like to customize the default import alias (@/*)? You can use the @ symbol instead of long URL chains on import statements or change the symbol.
After you answer the questions, the Next.js installer will create your project and initialize a new git repository. When it's done, cd into your new app.
$ cd my-app
Then, type code .
and VS Code will open a new window with the contents of the entire directory in the sidebar. Click Publish Branch to make your initial commit.

Now, open up the package.json
file. You'll see several scripts that you can run:
"scripts": {
"dev": "next dev",
"build": "next build",
"start": "next start",
"lint": "next lint"
},
Use the "dev" script to start your development server:
$ npm run dev
Next.js will automatically open up on localhost:3000
and your terminal should look like this:

You now have a Next.js application in Typescript with Tailwind CSS, ESLint, Prettier, and a git repo, just like that. What you decide to build is up to you. Jump into page.tsx
and get busy.

Happy coding! ✨
Please don't hesitate to share your thoughts and ideas with me. You can reach me on Twitter or find me on my other social media by clicking one of the links in the footer.